Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it is able to trap after raining.
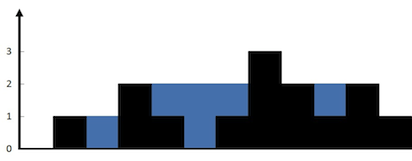
The above elevation map is represented by array [0,1,0,2,1,0,1,3,2,1,2,1]. In this case, 6 units of rain water (blue section) are being trapped. Thanks Marcos for contributing this image!
Example:
Input: [0,1,0,2,1,0,1,3,2,1,2,1] Output: 6
Java Solution :
class Solution {
public int trap(int[] height) {
int[] leftMax = new int[height.length];
int[] rightMax = new int[height.length];
int waterCollected = 0;
if(height == null || height.length == 0)
return 0;
// scan from left to right
leftMax[0] = height[0];
int max = leftMax[0];
for(int i =1 ;i < height.length; i++){
if(height[i] >= max){
max = height[i];
leftMax[i] = max;
} else{
leftMax[i] = max;
}
}
// scan from right to left
rightMax[height.length-1] = height[height.length-1];
int mr = rightMax[height.length-1];
for(int i = height.length-2; i >= 0 ; i--){
if(height[i] >= mr){
mr = height[i];
rightMax[i] = mr;
} else{
rightMax[i] = mr;
}
}
for(int i = 0 ;i < height.length; i++){
waterCollected += Math.min(leftMax[i],rightMax[i]) - height[i];
}
return waterCollected;
}
}