Serialization is the process of converting a data structure or object into a sequence of bits so that it can be stored in a file or memory buffer, or transmitted across a network connection link to be reconstructed later in the same or another computer environment.
Design an algorithm to serialize and deserialize a binary tree. There is no restriction on how your serialization/deserialization algorithm should work. You just need to ensure that a binary tree can be serialized to a string and this string can be deserialized to the original tree structure.
Clarification: The input/output format is the same as how LeetCode serializes a binary tree. You do not necessarily need to follow this format, so please be creative and come up with different approaches yourself.
Example 1:
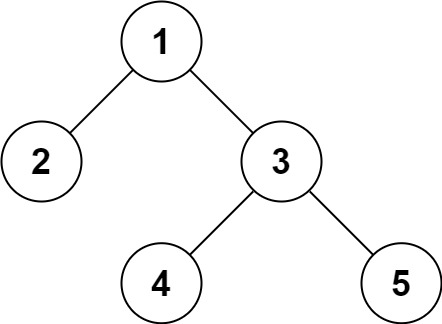
Input: root = [1,2,3,null,null,4,5] Output: [1,2,3,null,null,4,5]
Example 2:
Input: root = [] Output: []
Example 3:
Input: root = [1] Output: [1]
Example 4:
Input: root = [1,2] Output: [1,2]
class Codec: ''' O(n) time and O(n) space, BFS traversal e.g., 1 / \ 2 5 / \ 3 4 , level order traversal, serialize will be '1,2,5,3,4,None,None,None,None,None,None,'; deserialize with queue as well, convert back. Time and Space O(n). ''' def serialize(self, root): if not root: return '' queue = collections.deque() queue.append(root) res = '' while queue: node = queue.popleft() if not node: res += 'None,' continue res += str(node.val) + ',' queue.append(node.left) queue.append(node.right) return res def deserialize(self, data): if not data: return None ls = data.split(',') root = TreeNode(int(ls[0])) queue = collections.deque() queue.append(root) i = 1 while queue and i < len(ls): node = queue.popleft() if ls[i] != 'None': left = TreeNode(int(ls[i])) node.left = left queue.append(left) i += 1 if ls[i] != 'None': right = TreeNode(int(ls[i])) node.right = right queue.append(right) i += 1 return root # Your Codec object will be instantiated and called as such: # codec = Codec() # codec.deserialize(codec.serialize(root))
No comments:
Post a Comment